Creating custom rules for AutomateWoo can get complex very quickly, so we provide abstract rule classes for each rule type (string, number, object, select, date) that add helper methods.
Basic rule example
↑ Back to topFor the sake of simplicity, the example below doesn’t use one of the abstract classes. Below is a basic example of creating a rule that checks whether the billing post code of an order matches a supplied value.
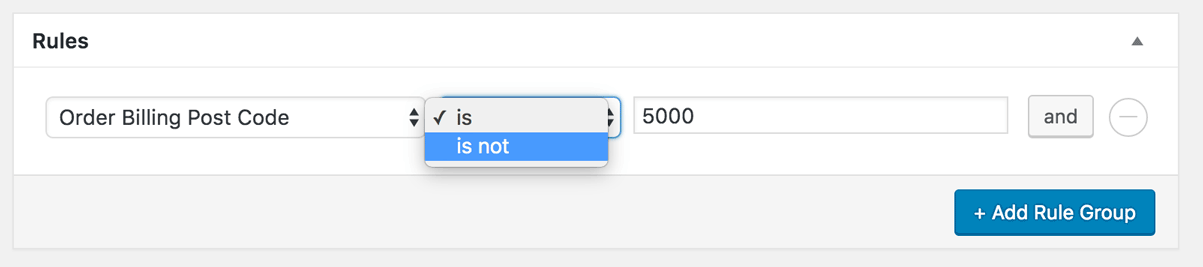
Date rule example
↑ Back to topThe following example shows how a custom field can be used to create a custom date rule. The advantage of creating a custom date rule is you can use AutomateWoo’s existing date based rule features which add more segmenting functionality over a standard custom field rule.
It’s important to note that the format of the date field should be one that PHP can understand.
